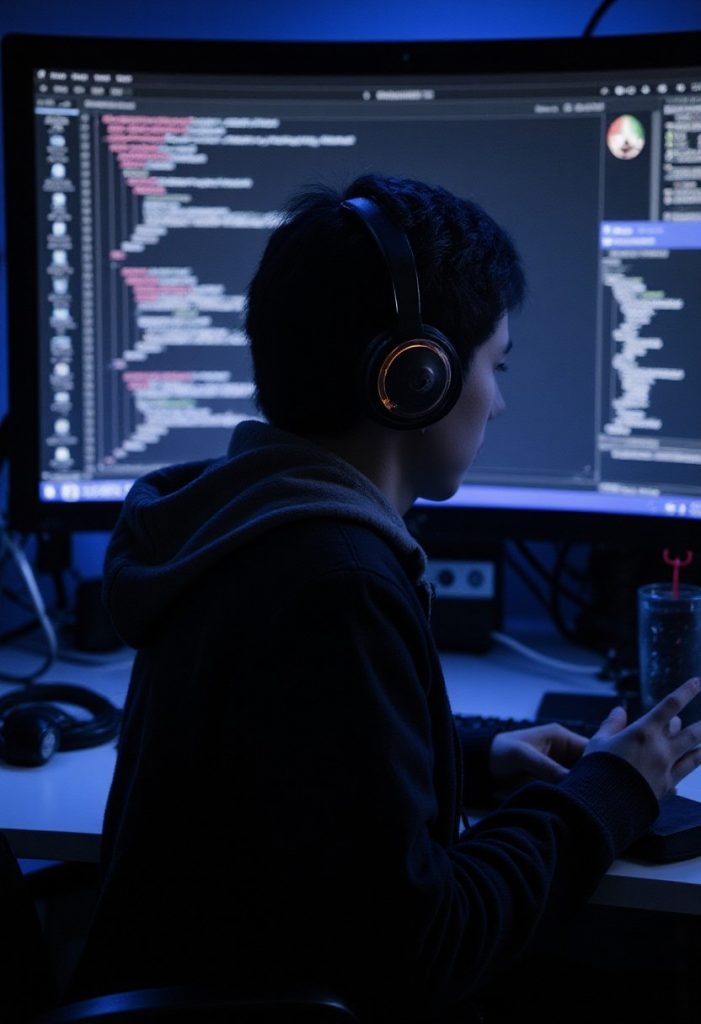
npm publish
First create an account: https://www.npmjs.com/signup.
Suppose you have a hello world package. Setup a github repo for it and include important instructions in the README.md. The most difficult part is setting up the configurations in your package.json and tsconfig.json. Before this you need to run
npm init
You will complete the following information:
package name
: This defaults to your directory name. Make sure it’s unique on npm and follows naming conventions (lowercase, no spaces, can use hyphens or underscores).version
: Defaults to 1.0.0. This follows semantic versioning (major.minor.patch).description
: A brief description of what your package does.entry point
: This is where your package’s main file lives. If you’re using TypeScript, you’ll want to change this later to point to your compiled JavaScript file (usually dist/index.js).test command
: The command to run your tests. You can leave this empty for now.git repository
: If you’re using git, provide the repository URL.keywords
: Words that help people find your package on npm.author
: Your name or organization name.license
: Defaults to ISC. You might want to use MIT or another license.
Assuming you want to use ES modules here are some examples to get you started:
// tsconfig.json
{
"compilerOptions": {
"target": "ESNext",
"module": "ESNext",
"declaration": true,
"declarationMap": true,
"sourceMap": true,
"outDir": "./dist",
"strict": true,
"removeComments": true,
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true,
"skipLibCheck": true
},
"include": ["*"],
"exclude": ["node_modules", "dist"]
}
Depending on your exact requirements you may need to add/remove or change some of these settings. You may want to choose a specific ES module version. Your package.json may include these settings:
// package.json
// ...
"main": "./dist/index.js",
"module": "./dist/index.js",
"types": "./dist/index.d.ts",
"exports": {
".": {
"import": "./dist/index.js",
"require": "./dist/index.js",
"types": "./dist/index.d.ts"
}
},
"scripts": {
"build": "tsc",
//...
These settings are helpful when you have a Typescript package that must be built (transpiled into Javascript).
Once your project is completed you can run
npm login
npm publish
–tag <tag name>
if the tag argument is omitted, by default it will latest
.